728x90
728x90
제네릭(Generic)
들어가며
- 타입스크립트(TypeScript)의 제네릭(Generic)에 대해 정리해본다.
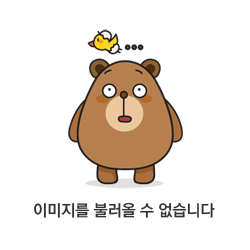
제네릭(Generic)
개념
- 코드의 재사용성을 높이고 다양한 타입에 대해 동작할 수 있게 해주는 기능
- 제네릭을 사용하면 타입을 우선 특정하지 않고, 나중에 사용할 때 그 타입을 지정할 수 있다.
- 또한, 타입 추론이 더 강화되고, 반복적인 타입 코드를 줄일 수 있다.
- 함수, 클래스, 인터페이스, 타입 등에 제네릭을 적용할 수 있다.
기본 문법
- 제네릭을 사용할 때는 타입 매개변수(Type Parameter)를 정의한다.
- 보통
<T>
와 같은 형식으로 사용하며, 원하는 이름을 지정하면 된다.
T
,K
,U
등이 관습적인 이름으로 쓰인다.
예제 코드 1 : 함수에서 제네릭 사용하기
identity
함수는 입력받는 값의 타입에 상관없이 같은 타입의 값을 반환한다.
function identity<T>(arg: T): T { return arg; } const result1 = identity<string>("Hello"); // string const result2 = identity<number>(100); // number
예제 코드 2 : 배열 타입에서 제네릭 사용하기
T[]
는 제네릭 배열로, 배열의 요소 타입을 제네릭 타입으로 받는다.
function logArrayLength<T>(arr: T[]): void { console.log(arr.length); } logArrayLength<number>([1, 2, 3]); // 숫자 배열 logArrayLength<string>(["a", "b", "c"]); // 문자열 배열
사용하기
제네릭 인터페이스
- 인터페이스(Interface)에도 제네릭을 적용할 수 있다.
interface Box<T> { value: T; } const stringBox: Box<string> = { value: "Hello" }; const numberBox: Box<number> = { value: 100 };
⇒ Box<T>
는 제네릭 인터페이스로, T
타입을 받는 value
속성을 정의한다.
제네릭 클래스
- 클래스(Class)에도 제네릭을 사용할 수 있다.
class Container<T> { private _content: T; constructor(value: T) { this._content = value; } get content(): T { return this._content; } } const stringContainer = new Container<string>("Hello"); console.log(stringContainer.content); // "Hello" const numberContainer = new Container<number>(123); console.log(numberContainer.content); // 123
제약조건(Constraints)
- 제네릭 타입에 특정 조건을 걸고 싶을 때는
extends
키워드를 사용한다.
function getLength<T extends { length: number }>(item: T): number { return item.length; } getLength("Hello"); // 문자열은 length 속성을 가지고 있으므로 오류가 발생하지 않는다. getLength([1, 2, 3]); // 배열도 length 속성을 가지고 있으므로 오류가 발생하지 않는다. // getLength(123); // 오류 (number 타입은 length 속성이 없다.)
⇒ T extends { length: number }
는 length
속성을 가진 타입만 받을 수 있도록 제약을 건 것이다.
참고 사이트
Documentation - Generics
Types which take parameters
www.typescriptlang.org
728x90
728x90
'Programming > TypeScript' 카테고리의 다른 글
[TypeScript] 환경 변수 타입 설정하기 (3) | 2024.12.09 |
---|---|
[TypeScript] 클래스(Class) (0) | 2024.10.12 |
[TypeScript] 인터페이스(Interface) (0) | 2024.10.12 |
[TypeScript] Zod 라이브러리 (0) | 2024.10.11 |
[TypeScript] 타입 가드(Type Guard) (0) | 2024.10.10 |
[TypeScript] 모듈 방식 사용하기 (0) | 2024.10.10 |
[TypeScript] 인터페이스(Interface)와 타입 별칭(Type Alias) 비교 (0) | 2024.10.09 |
[TypeScript] ! 연산자(Non-null Assertion Operator) (0) | 2024.08.20 |