728x90
728x90
카메라 권한 요청 메시지 표시 방법 (Expo)
들어가며
- 엑스포(Expo)를 이용하여 생성한 리액트 네이티브(React Native) 프로젝트에서 카메라 권한 요청 메시지를 표시하는 방법을 정리해본다.
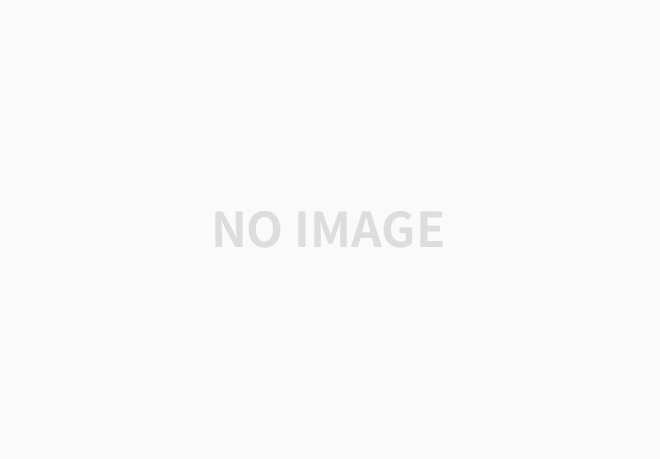
방법
패키지 설치
- 터미널에서 아래 명령을 실행하여
expo-camera
패키지를 설치해준다.
$ npx expo install expo-camera
앱 설정 파일 구성하기 (app.json
)
- 프로젝트의 최상단에 있는 앱 설정 파일(
app.json
)에 아래의 내용을 추가해준다.
/app.json
{ "expo": { "plugins": [ [ "expo-camera", { "cameraPermission": "Allow $(PRODUCT_NAME) to access your camera", "microphonePermission": "Allow $(PRODUCT_NAME) to access your microphone", "recordAudioAndroid": true } ] ] } }
옵션 설명
옵션 | 기본값 | 설명 |
cameraPermission |
"Allow $(PRODUCT_NAME) to access your camera" |
▶iOS - 카메라 사용 권한 허용 요청 메시지 |
microphonePermission |
"Allow $(PRODUCT_NAME) to access your microphone" |
▶ iOS - 마이크 사용 권한 허용 요청 메시지 설정 |
recordAudioAndroid |
true |
▶ Android - 안드로이드 기기에서 오디오 녹음 권한을 허용할 지의 여부 - true 로 설정 시, 오디오 녹음을 위해 해당 권한을 요청하게 되며, false 로 설정하면 오디오 권한을 사용하지 않는다. |
⇒ $(PRODUCT_NAME)
는 앱 이름으로 자동 치환된다.
사용하기
- 크게 ①카메라 권한 요청 전 화면을 보여주는 코드와, ②카메라 권한이 승인되지 않았을 때 화면을 보여주는 코드를 추가한다.
useCameraPermissions
훅을 이용하여 카메라 권한 승인 요청을 하는 함수(requestPermission
)을 불러와서 사용한다.- 이 함수를 권한 승인 요청 컴포넌트의
onPress
속성의 값으로 넣어준다.
- 이 함수를 권한 승인 요청 컴포넌트의
import { CameraView, CameraType, useCameraPermissions } from 'expo-camera'; import { useState } from 'react'; import { Button, StyleSheet, Text, TouchableOpacity, View } from 'react-native'; export default function App() { const [permission, requestPermission] = useCameraPermissions(); // (1) 빈 화면 표시 (카메라 권한 요청 전 화면, 보통 로딩 스피너를 넣어준다.) if (!permission) { // Camera permissions are still loading. return <View />; } // (2) 카메라 사용 권한이 승인되지 않았을 경우의 화면 표시 if (!permission.granted) { return ( <View > <Text>We need your permission to show the camera</Text> <Button onPress={requestPermission} title="grant permission" /> </View> ); } // ... }
사용 예시 코드
import { CameraView, CameraType, useCameraPermissions } from 'expo-camera'; import { useState } from 'react'; import { Button, StyleSheet, Text, TouchableOpacity, View } from 'react-native'; export default function App() { const [facing, setFacing] = useState<CameraType>('back'); const [permission, requestPermission] = useCameraPermissions(); if (!permission) { // Camera permissions are still loading. return <View />; } if (!permission.granted) { // Camera permissions are not granted yet. return ( <View style={styles.container}> <Text style={styles.message}>We need your permission to show the camera</Text> <Button onPress={requestPermission} title="grant permission" /> </View> ); } function toggleCameraFacing() { setFacing(current => (current === 'back' ? 'front' : 'back')); } return ( <View style={styles.container}> <CameraView style={styles.camera} facing={facing}> <View style={styles.buttonContainer}> <TouchableOpacity style={styles.button} onPress={toggleCameraFacing}> <Text style={styles.text}>Flip Camera</Text> </TouchableOpacity> </View> </CameraView> </View> ); } const styles = StyleSheet.create({ container: { flex: 1, justifyContent: 'center', }, message: { textAlign: 'center', paddingBottom: 10, }, camera: { flex: 1, }, buttonContainer: { flex: 1, flexDirection: 'row', backgroundColor: 'transparent', margin: 64, }, button: { flex: 1, alignSelf: 'flex-end', alignItems: 'center', }, text: { fontSize: 24, fontWeight: 'bold', color: 'white', }, });
참고 사이트
Camera
A React component that renders a preview for the device's front or back camera.
docs.expo.dev
728x90
728x90
'Programming > React Native' 카테고리의 다른 글
[React Native] SafeAreaView (0) | 2025.04.03 |
---|---|
[React Native] Alert API (0) | 2025.04.03 |
[React Native] npm install vs. expo install (0) | 2025.04.03 |
[React Native] 환경 변수 파일 사용하기 (react-native-dotenv) (0) | 2025.04.03 |
[React Native] 클립보드 기능 구현하기 (expo-clipboard) (0) | 2025.02.24 |
[React Native] blurOnSubmit 속성과 submitBehavior 속성 (0) | 2025.02.23 |
[React Native] TailwindCSS IntelliSense 활성화하기 (VS Code) (0) | 2025.02.19 |
[React Native] 클릭 시, 리플 효과(Ripple Effect) 주는 방법 (1) | 2024.12.11 |