React Hook Form 라이브러리
들어가며
- 리액트(React.js) 애플리케이션에서 폼(Form)의 상태 관리를 쉽게 해주는 라이브러리인 React Hook Form에 대해 정리해본다.
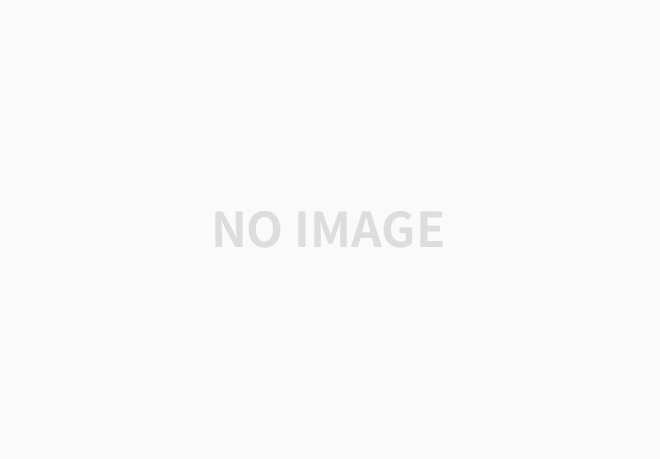
React Hook Form
개념
- 리액트 애플리케이션에서 폼(Form)의 상태를 관리해주는 라이브러리
- 간단하고 효율적이며 퍼포먼스가 뛰어나다.
- 리액트의 훅(Hook)을 활용하여 사용하며, 폼 상태 관리를 위한 기본적인 기능과 유효성 검증(Validation) 기능을 제공한다.
React Hook Form - performant, flexible and extensible form library
Performant, flexible and extensible forms with easy-to-use validation.
react-hook-form.com
설치
$ npm install react-form-hook # yarn add react-form-hook
주요 특징
- 직관적이고 배우기 쉬운 API로 폼 상태 관리를 간단하게 수행할 수 있다.
- 비제어 컴포넌트(Uncontrolled Component)를 사용하여 리렌더링을 최소화하고 성능을 최적화한다.
- 비제어 컴포넌트(Uncontrolled Component)
- 리액트에서 폼 요소의 값을 리액트 상태(State)로 관리하지 않고, DOM 자체에서 값을 관리하는 방식으로 작동하는 컴포넌트
- 리액트의 상태(State)가 아닌, 기본 HTML 폼 요소의 상태를 직접적으로 사용하는 방식
- 리액트에서 폼 요소의 값을 리액트 상태(State)로 관리하지 않고, DOM 자체에서 값을 관리하는 방식으로 작동하는 컴포넌트
- 비제어 컴포넌트(Uncontrolled Component)
- 다양한 유효성 검증을 쉽게 설정할 수 있으며, 기본 HTML5 유효성 검증 속성을 지원한다.
- 외부 라이브러리(
yup
또는zod
)와 통합 가능하다.
- 외부 라이브러리(
- 다른 폼 관리 라이브러리보다 가볍고 빠르다.
사용하기
- React Hook Form은
useForm
훅을 기본으로 작동한다. - 예시 코드와 함께 사용하는 방법을 정리해본다.
폼 생성하기
App.tsx
import { useForm } from 'react-hook-form'; type FormValues = { username: string; email: string; }; const App = () => { const { register, // 폼 입력을 등록하는 메서드 handleSubmit, // 폼 제출 처리 formState: { isSubmitting, isDirty, touchedFields, errors }, // 폼의 유효성 검사 상태 } = useForm<FormValues>(); const onSubmit = (data: FormValues) => { console.log(data); }; return ( <form onSubmit={handleSubmit(onSubmit)}> <div> <label>Username:</label> <input {...register("username", { required: "Username is required" })} /> {errors.username && <p>{errors.username.message}</p>} </div> <div> <label>Email:</label> <input {...register("email", { required: "Email is required", pattern: { value: /^[^]+[^]+\.[^]+$/, message: "Invalid email address" } })} /> {errors.email && <p>{errors.email.message}</p>} </div> <button type="submit" disabled={isSubmitting}> {isSubmitting ? "Submitting..." : "Submit"} </button> </form> ); }; export default App;
유효성 검증
<input {...register("password", { required: true, minLength: 8 })} />
register
함수의 2번째 인자로 유효성 검증 규칙을 전달할 수 있다.
유효성 검증 규칙 속성
속성 | 설명 |
required |
필수 입력 값 |
maxLength / minLength |
입력 길이 제한 |
pattern |
정규식 패턴 |
validate |
커스텀 검증 로직 |
폼 상태 관리
const { register, handleSubmit, formState: { isSubmitting, isDirty, touchedFields, errors }, } = useForm<FormValues>();
- React Hook Form의
formState
를 통해 폼의 다양한 상태를 확인할 수 있다.
formState
속성
속성 | 설명 |
isSubmitting |
폼이 제출 중인지 확인 |
isDirty |
입력값이 변경되었는지 확인 |
touchedFields |
포커스 설정 후 해제된 필드 목록 |
errors |
유효성 검사 에러 정보 |
외부 라이브러리와의 통합
- React Hook Form은
yup
또는zod
같은 스키마 기반 유효성 검증 라이브러리와 쉽게 통합된다.
예시 코드
import { useForm } from 'react-hook-form'; import { yupResolver } from '@hookform/resolvers/yup'; import * as yup from 'yup'; const schema = yup.object({ username: yup.string().required("Username is required"), email: yup.string().email("Invalid email").required("Email is required"), }).required(); const App = () => { const { register, handleSubmit, formState: { errors }, } = useForm({ resolver: yupResolver(schema), // yup 스키마를 유효성 검증 규칙으로 사용 }); const onSubmit = (data) => { console.log(data); }; return ( <form onSubmit={handleSubmit(onSubmit)}> <input {...register("username")} /> <p>{errors.username?.message}</p> <input {...register("email")} /> <p>{errors.email?.message}</p> <button type="submit">Submit</button> </form> ); }; export default App;
React Hook Form과 컨트롤러
- React Hook Form은 제어 컴포넌트(Controlled Components)도 지원한다.
- 이때,
<Controller>
컴포넌트를 사용한다.
예시 코드
import { useForm, Controller } from 'react-hook-form'; import Select from 'react-select'; const App = () => { const { control, handleSubmit } = useForm(); const onSubmit = (data) => { console.log(data); }; return ( <form onSubmit={handleSubmit(onSubmit)}> <Controller name="select" control={control} defaultValue="" render={({ field }) => <Select {...field} options={[{ value: '1', label: 'Option 1' }]} />} /> <button type="submit">Submit</button> </form> ); }; export default App;
주요 훅(Hook)
- React Hook Form의 주요 훅(Hook)으로
useForm
,useFormContext
,useWatch
,useFieldArray
등이 있다.
훅 | 설명 |
useForm |
폼 생성 및 상태 관리 |
useFormContext |
폼 상태를 컨텍스트로 공유 |
useWatch |
특정 입력값 변화 감지 |
useFieldArray |
필드 배열 관리 (동적 입력 필드) |
참고 사이트
React Hook Form - performant, flexible and extensible form library
Performant, flexible and extensible forms with easy-to-use validation.
react-hook-form.com
react-hook-form
Performant, flexible and extensible forms library for React Hooks. Latest version: 7.53.2, last published: 15 days ago. Start using react-hook-form in your project by running . There are 5543 other projects in the npm registry using
www.npmjs.com
'Programming > React' 카테고리의 다른 글
[React.js] 폼(Form) 처리 방법 (React 19) (0) | 2025.01.21 |
---|---|
[React.js] 객체 표기법(Object Notation) 방식과 빌더 콜백 표기법(Builder Callback Notation) 방식 (0) | 2024.11.13 |
[React.js] .js 파일에서 Uncaught SyntaxError: Unexpected token '<' 오류 발생할 때 해결 방법 (Vite) (0) | 2024.11.13 |
[React.js] <Link> 컴포넌트와 <NavLink> 컴포넌트 비교 (React Router) (0) | 2024.11.13 |
[React.js] 리덕스(Redux), 리덕스 툴킷(Redux Toolkit) (0) | 2024.11.11 |
[React.js] 모든 웹 브라우저에서 공통된 HTML 요소 스타일이 보여지도록 설정하는 방법 (normalize.css) (0) | 2024.11.07 |
[React.js] Recharts 라이브러리 (1) | 2024.11.06 |
[React.ts] PropsWithChildren (0) | 2024.11.05 |