728x90
728x90
JSON(JavaScript Object Notation) 다루기
들어가며
- 웹 서버 뿐만 아니라 다양한 곳에서 데이터를 교환할 때 사용되는 파일 형식인 JSON(JavaScript Object Notation)에 대해 정리해본다.
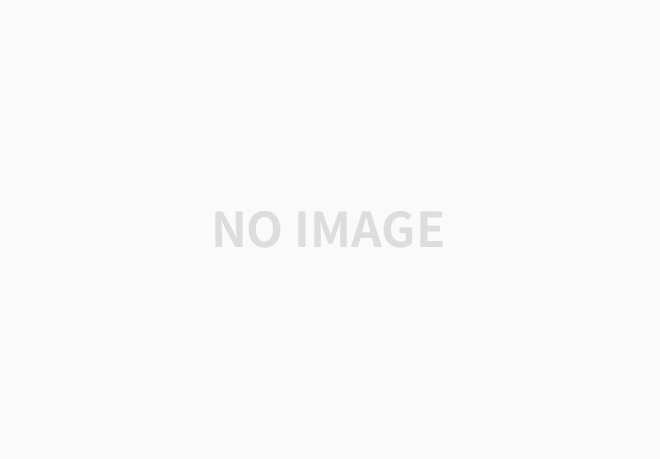
JSON(JavaScript Object Notation)
개념
- 데이터 교환 형식으로 널리 사용되며, 문자열로 인코딩된 객체를 포함한 구조화된 데이터를 표현한다.
- 자바스크립트는 JSON 데이터를 다루기 위해 JSON 객체를 제공한다.
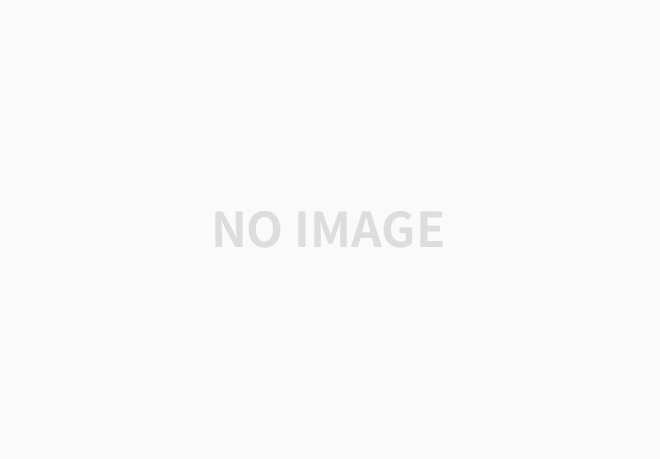
주요 메서드
- JSON 객체의 주요 메서드로는
JSON.stringify()
와JSON.parse()
가 있다.
① JSON.stringfy()
- 자바스크립트 객체(
{ prop: value, ... }
)나 배열([value1, ..., valueN]
)을 JSON 문자열로 변환한다.
JSON.stringify(value, replacer, space)
매개변수 | 설명 |
value | JSON 문자열로 변환할 자바스크립트 값 (객체, 배열 등) [필수] |
replacer | JSON 문자열로 변환하는 과정에서 특정 속성을 필터링하거나 수정하는 함수 또는 배열 [선택] |
space | 출력 JSON 문자열의 들여쓰기 수준을 설정하는 숫자나 문자열 [선택] |
사용 예제
const obj = { name: "John", age: 30, city: "New York" }; const jsonString = JSON.stringify(obj); console.log(jsonString); // {"name":"John","age":30,"city":"New York"} // (1) replacer를 사용할 경우 const replacer = (key, value) => { if (typeof value === "string") { return undefined; } return value; }; const jsonStringWithReplacer = JSON.stringify(obj, replacer); console.log(jsonStringWithReplacer); // {"age":30} // (2) space를 사용할 경우 const jsonStringWithSpace = JSON.stringify(obj, null, 2); console.log(jsonStringWithSpace); /* { "name": "John", "age": 30, "city": "New York" } */
JSON.stringfy() : JS 객체/배열 → JSON
② JSON.parse()
- JSON 문자열을 자바스크립트 객체나 배열로 변환한다.
JSON.parse(text, receiver)
매개변수 | 설명 |
text | 자바스크립트 객체나 배열로 변환할 JSON 문자열 [필수] |
receiver | 변환된 결과 객체의 각 속성을 처리하는 함수 [선택] |
사용 예제
const jsonString = '{"name":"John","age":30,"city":"New York"}'; const obj = JSON.parse(jsonString); console.log(obj); // { name: 'John', age: 30, city: 'New York' } // receiver를 사용할 경우 const reviver = (key, value) => { if (key === "age") { return value + 1; } return value; }; const objWithReviver = JSON.parse(jsonString, reviver); console.log(objWithReviver); // { name: 'John', age: 31, city: 'New York' }
JSON.parse(json_value) : JSON → JS 객체/배열
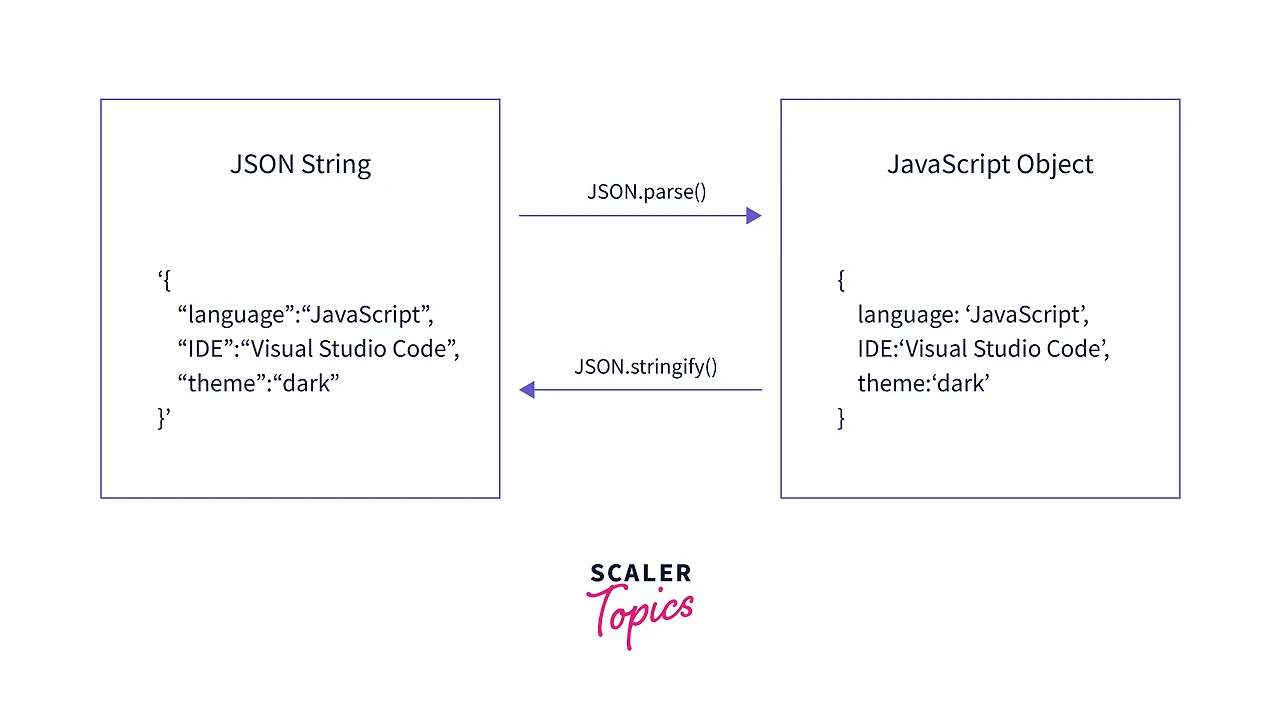
참고 사이트
JSON - JavaScript | MDN
JSON 객체는 JavaScript Object Notation(JSON)을 분석하거나 값을 JSON으로 변환하는 메서드를 가지고 있습니다. JSON을 직접 호출하거나 인스턴스를 생성할 수 없으며, 두 개의 메서드를 제외하면 자신만의
developer.mozilla.org
728x90
728x90
'Programming > JavaScript' 카테고리의 다른 글
[JavaScript] Faker.js 라이브러리 (0) | 2024.10.30 |
---|---|
[JavaScript] fetch() API와 Axios의 에러 처리 방법 비교 (0) | 2024.08.30 |
[JavaScript] 전개 연산자 (Spread Operator, ...) (0) | 2024.08.27 |
[JavaScript] for 문 정리 (for, for...in, for...of, forEach, for await...of) (0) | 2024.08.25 |
[JavaScript] Intl.NumberFormat 객체 (0) | 2024.06.28 |
[JavaScript] 옵셔널 체이닝 연산자(Optional Chaining Operator), null 병합 연산자(Nullish Coalescing Operator) (ES11(ECMAScript2020)) (0) | 2024.05.16 |
[JavaScript] 비동기 프로그래밍(Asynchronous Programming) (0) | 2024.01.24 |
[JavaScript] 변수 재선언과 재할당 (var, let, const) (1) | 2024.01.18 |