728x90
728x90
useImperativeHandle과 forwardRef
들어가며
- 리액트(React.js)에서 사용되는
useImperativeHandle
과forwardRef
에 대해 알아보자. forwardRef
와useImperativeHandle
은 컴포넌트 간의 참조를 다루기 위한 도구이다.- 이 두가지 개념을 이해하면 컴포넌트 외부에서 내부의 DOM 요소나 메서드에 접근할 수 있게 되어, 고급 컴포넌트 설계에 유용하다고 한다.
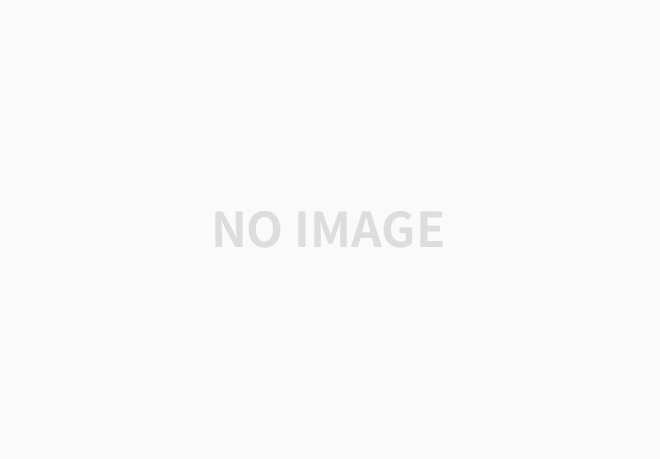
forwardRef
개념
- 부모 컴포넌트로부터 전달된
ref
를 자식 컴포넌트가 받을 수 있게 해준다.forwardRef
를 사용하지 않고 자식 컴포넌트에ref
를 전달할 수 없다.ref
는 DOM 요소에만 붙일 수 있다.
- 하지만
forwardRef
를 사용하면, 부모 컴포넌트가 자식 컴포넌트의 DOM 요소나 인스턴스에 접근할 수 있다.
사용법
부모 컴포넌트
- 자식 컴포넌트(
MyInput
)의 인자로ref
를 넘겨주는 모습이다.
import React, { useRef } from 'react'; import MyInput from './MyInput'; const ParentComponent = () => { const inputRef = useRef(); const focusInput = () => { inputRef.current.focus(); }; return ( <div> <MyInput ref={inputRef} /> <button onClick={focusInput}>Focus the input</button> </div> ); }; export default ParentComponent;
자식 컴포넌트
import React, { forwardRef } from 'react'; const MyInput = forwardRef((props, ref) => ( <input ref={ref} {...props} /> )); export default MyInput;
useImperativeHandle
개념
forwardRef
와 함께 사용되어, 부모 컴포넌트가 자식 컴포넌트의 특정 기능에 접근할 수 있게 해준다.- 부모 컴포넌트가 자식 컴포넌트의 내부 구현을 몰라도 (자식 컴포넌트의) 특정 메서드를 호출할 수 있다.
사용법
부모 컴포넌트
- 자식 컴포넌트(
customInput
) 안의clear
메서드를 부모 컴포넌트(ParentComponent
)에서 사용할 수 있다.
import React, { useRef } from 'react'; import CustomInput from './CustomInput'; const ParentComponent = () => { const customInputRef = useRef(); const handleFocus = () => { customInputRef.current.focus(); }; const handleClear = () => { customInputRef.current.clear(); }; return ( <div> <CustomInput ref={customInputRef} /> <button onClick={handleFocus}>Focus the input</button> <button onClick={handleClear}>Clear the input</button> </div> ); }; export default ParentComponent;
자식 컴포넌트
import React, { useRef, useImperativeHandle, forwardRef } from 'react'; const CustomInput = forwardRef((props, ref) => { const inputRef = useRef(); useImperativeHandle(ref, () => ({ focus: () => { inputRef.current.focus(); }, clear: () => { inputRef.current.value = ''; } })); return <input ref={inputRef} {...props} />; }); export default CustomInput;
정리
forwardRef
는 부모 컴포넌트가 자식 컴포넌트의 DOM 요소나 인스턴스에 접근할 수 있게 해준다.useImperativeHandle
는 자식 컴포넌트에서 부모 컴포넌트가 접근할 수 있는 메서드를 정의할 수 있게 해준다.
참고 사이트
useImperativeHandle – React
The library for web and native user interfaces
react-ko.dev
forwardRef – React
The library for web and native user interfaces
react-ko.dev
728x90
728x90
'Programming > React' 카테고리의 다른 글
[React.js] useEffect와 useCallback (0) | 2024.06.24 |
---|---|
[React.js] useReducer 훅 (0) | 2024.06.07 |
[React.js] map 함수를 사용할 때 중괄호({})와 소괄호(()) (0) | 2024.05.29 |
[React.js] 토스트 메시지 띄우기 간단 예제 (ReactDOM.createPortal) (0) | 2024.05.21 |
[React.js] 부모 컴포넌트에서 자식 컴포넌트로 요소 넘기는 방법 (0) | 2024.05.14 |
[React.js] 실시간으로 특정 요소의 길이값 가져오기 (0) | 2024.03.26 |
[React.js] state와 ref 비교하기 (0) | 2024.03.02 |
[React.js] 마우스 호버 효과를 적용하기 위한 커스텀 훅 만들기 (useHover.js) (0) | 2024.02.20 |