728x90
문제
두 정수 A와 B를 입력받은 다음, A/B를 출력하는 프로그램을 작성하시오.
입력
첫째 줄에 A와 B가 주어진다. (0 < A, B < 10)
출력
첫째 줄에 A/B를 출력한다. 실제 정답과 출력값의 절대오차 또는 상대오차가 `10^{-9}` 이하이면 정답이다.
예제 입력 1
1 3
예제 출력 1
0.33333333333333333333333333333333
`10^{-9}` 이하의 오차를 허용한다는 말은 꼭 소수 9번째 자리까지만 출력하라는 뜻이 아니다.
예제 입력 2
4 5
예제 출력 2
0.8
출처
- 문제를 만든 사람: baekjoon
- 빠진 조건을 찾은 사람: djm03178
- 내용을 추가한 사람: jh05013
알고리즘 분류
- 수학
- 구현
- 사칙연산
문제 출처
https://www.acmicpc.net/problem/1008
1008번: A/B
두 정수 A와 B를 입력받은 다음, A/B를 출력하는 프로그램을 작성하시오.
www.acmicpc.net
문제 해결 방법
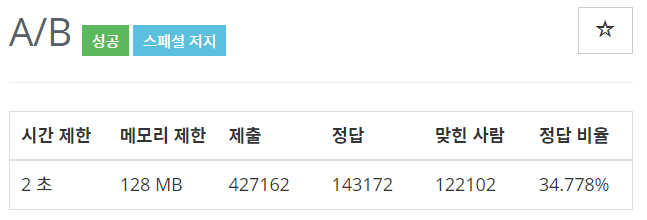
- 자료형에 대해 자세히 알 수 있어야 풀 수 있는 문제였다.
- `10^{-9}` 자리까지 정확성을 보장하는 부동 소수점 자료형을 사용해 문제를 해결하였다.
- float 형을 사용할 경우, `10^{-7}` 자리까지 정확한 값이 보장되므로 오답이다.
- double 형을 사용할 경우, `10^{-15}` 자리까지 정확한 값이 보장되므로 정답이다.
- long double 형을 사용할 경우, `10^{-18}` 자리까지 정확한 값이 보장되므로 정답이다.
0.33333334326744079589843750000000 // 1/3의 결과 (Float 형)
0.33333333333333331482961625624739 // 1/3의 결과 (Double 형)
0.33333333333333333334236835143738 // 1/3의 결과 (Long Double 형)
코드
#include <iostream>
using namespace std;
int main() {
cin.tie(NULL);
cout.tie(NULL);
ios_base::sync_with_stdio(false);
// Fix Precision
cout << fixed;
cout.precision(32);
double a, b; // or long double
cin >> a >> b;
cout << a / b << endl;
return 0;
}
채점 결과
참고
- [단계별로 풀어보기] > [입출력과 사칙연산]
C++ 에서의 자료형(Data Type)
- C++ 의 자료형에는 기본형, 문자형, 정수형, 실수형(부동 소수점형)이 있다.
- 기본형
- void
- 문자형
- (singed) char
- unsigned char
- wchar_t
- 정수형
- bool
- (signed) short (int)
- unsigned short (int)
- (signed) int
- unsigned int
- (signed) long (int)
- unsigned long (int)
- __int8
- __int16
- __int32
- __int64
- 실수형(부동 소수점형)
- float
- (long) double
- 기본형
정수 자료형의 크기 및 범위 (LLP64/IL32P64, Windows)
자료형 | 크기 | 범위 | 비고 |
char signed char |
1 Byte 8 Bits |
-128 ~ 127 | |
unsigned char | 1 Byte, 8 Bits | 0 ~ 255 | |
short short int |
2 Bytes 16 Bits |
-32,768 ~ 32,767 | int 생략 가능 |
unsigned short unsigned short int |
2 Bytes 16 Bits |
0 ~ 65,535 | int 생략 가능 |
int signed int |
4 Bytes 32 Bits |
-2,147,483,648 ~ 2,147,483,647 | |
unsigned unsigned int |
4 Bytes 32 Bits |
0 ~ 4,294,967,295 | int 생략 가능 |
long long int signed long signed long int |
4 Bytes 32 Bits |
-2,147,483,648 ~ 2,147,483,647 | int 생략 가능 |
unsigned long unsigned long int |
4 Bytes 32 Bits |
0 ~ 4,294,967,295 | int 생략 가능 |
long long long long int signed long long signed long long int |
8 Bytes 64 Bits |
-9,223,372,036,854,775,808 ~ 9,223,372,036,854,775,807 | int 생략 가능 |
unsigned long long unsigned long long int |
8 Bytes 64 Bits |
0 ~ 18,446,744,073,709,551,615 | int 생략 가능 |
자료형의 크기 및 범위 (Microsoft Visual C++)
Type Name | Bytes | Other Names | Range of Values |
int | * | signed signed int |
System Dependent |
unsigned int | * | unsigned | System Dependent |
__int8 | 1 | char signed char |
-128 to 127 |
__int16 | 2 | short short int signed short int |
–32,768 to 32,767 |
__int32 | 4 | signed signed int |
–2,147,483,648 to 2,147,483,647 |
__int64 | 8 | none | –9,223,372,036,854,775,808 to 9,223,372,036,854,775,807 |
bool | 1 | none | false or true |
char | 1 | signed char | –128 to 127 |
unsigned char | 1 | none | 0 to 255 |
long | 4 | long int signed long int |
–2,147,483,648 to 2,147,483,647 |
long long | 8 | none (but equivalent to __int64) |
–9,223,372,036,854,775,808 to 9,223,372,036,854,775,807 |
unsigned long | 4 | unsigned long int | 0 to 4,294,967,295 |
unsigned long long | 8 | unsigned long long int | 0 to 18,446,744,073,709,551,615 |
enum | * | none | Same as int |
float | 4 | none | 3.4E +/- 38 (7 digits) |
double | 8 | none | 1.7E +/- 308 (15 digits) |
long double | 8 | none | same as double |
wchar_t | 2 | __wchar_t | 0 to 65,535 |
※ float 형은 소수점 최대 7자리까지, double 형, long double 형은 소수점 최대 15자리까지의 정확성을 보장한다.
※ A variable of __wchar_t designates a wide-character or multibyte character type.
※ By default wchar_t is a typedef for unsigned short.
※ 참고 사이트 : click
소수점 이하 자릿수 개수 조절하기
- 다음과 같이 cout 으로 출력할 때, 원하는 만큼 소숫점 이하의 수가 출력되도록 할 수 있다.
cout << fixed;
cout.precision(number);
728x90
'Problem Solving > BOJ' 카테고리의 다른 글
[BOJ-10430][C++] 나머지 (0) | 2022.07.07 |
---|---|
[BOJ-18108][C++] 1998년생인 내가 태국에서는 2541년생? (0) | 2022.07.07 |
[BOJ-10926][C++] ??! (0) | 2022.07.07 |
[BOJ-10869][C++] 사칙연산 (0) | 2022.07.07 |
[BOJ-10998][C++] A×B (0) | 2022.07.07 |
[BOJ-1001][C++] A-B (0) | 2022.07.07 |
[BOJ-1000][C++] A+B (0) | 2022.07.07 |
[BOJ-10172][C++] 개 (0) | 2022.07.07 |